Easier account abstraction in Connect SDK (React)
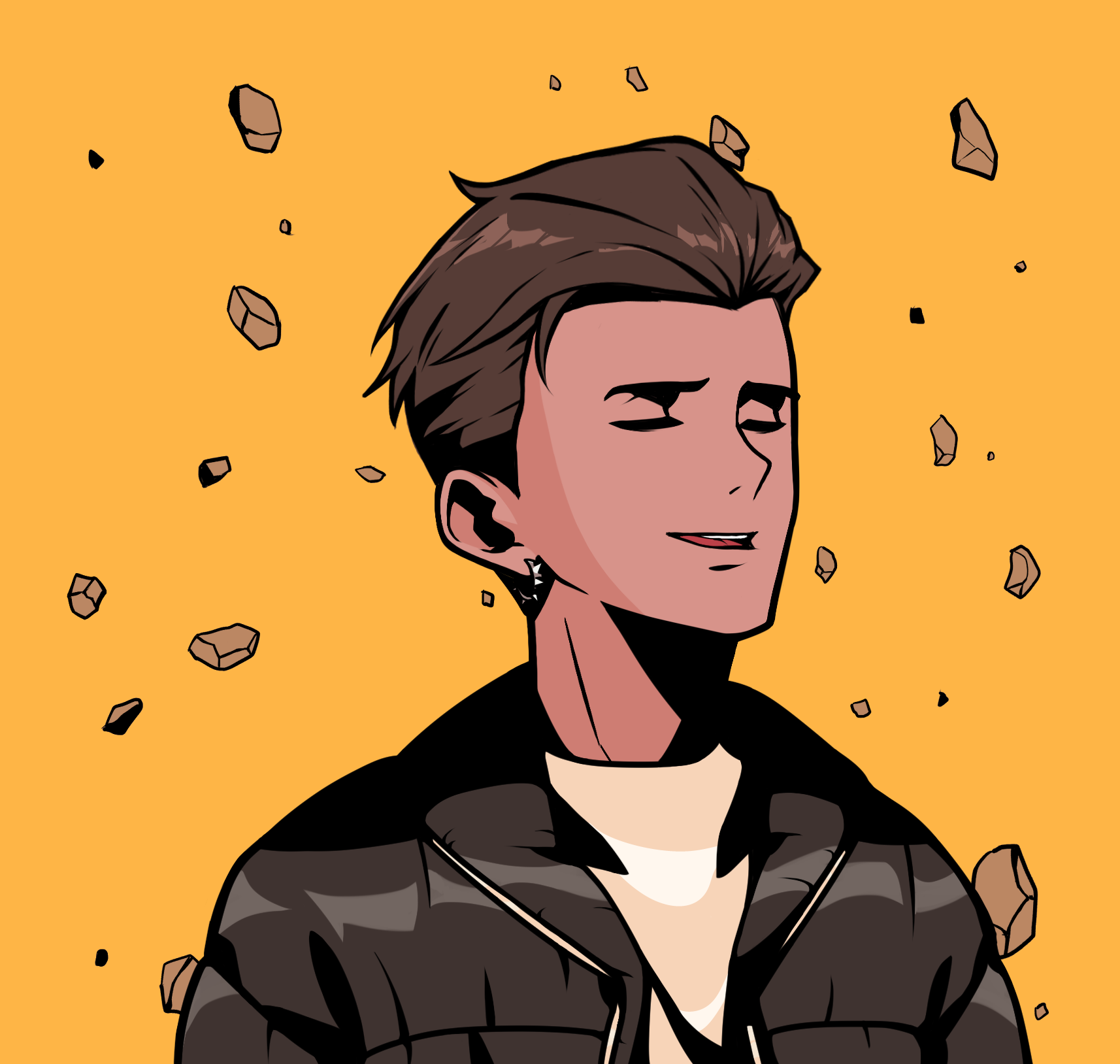
In the latest version of the Connect SDK (v5.12.0) we've made it easier to convert any EOA wallet to a smart account!
You can now pass the accountAbstraction
prop directly to useConnect
- this will ensure that any wallet connected via this hook will be converted to a smart account.
And of course, you can also use the ConnectButton
or ConnectEmbed
UI components and pass the accountAbstraction
prop to achieve the same thing with our prebuilt UI.
For more resources, check out the documentation.