Wallet Call API (EIP-5792) support in the Connect SDK
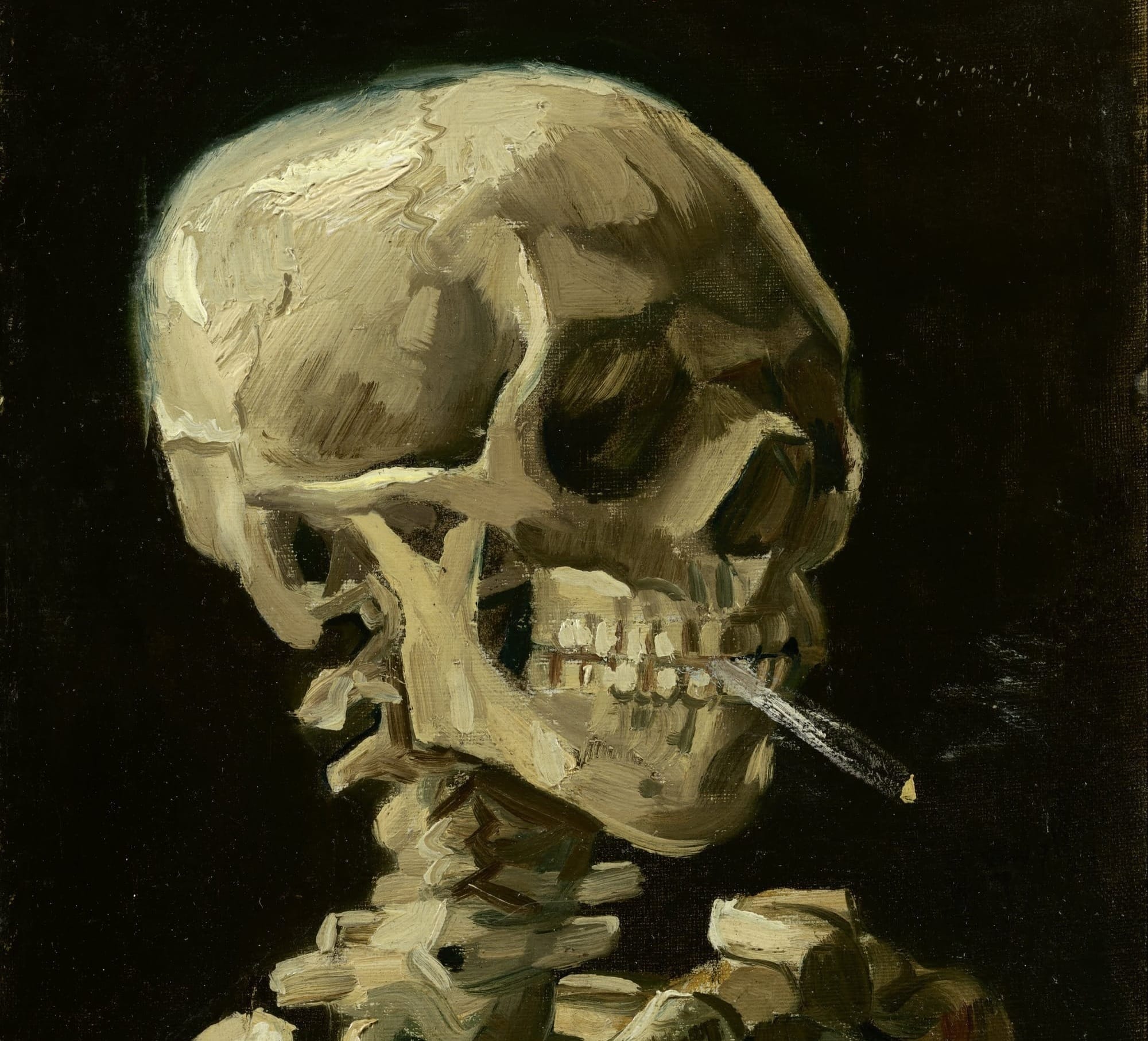
EIP-5792 simplifies how apps can leverage wallets' full capabilities like batch transactions, sponsored transactions, auxiliary funds, and anything else smart contract accounts are now capable of. We've added built-in support for wallet_sendCalls
, wallet_getCapabilities
, and wallet_getCallsStatus
so app developers can provide the best possible user experience regardless of the wallets their users bring to the app. With v5.22.0 the Connect SDK exposes 3 simple functions to take advantage of the Wallet Call API.
Send multiple transactions at once with any wallet
Send any number of transactions via the sendCalls
function. You prepare the transactions as if you were using sendTransactions
, then pass them as an array to sendCalls
.
We try to make sendCalls
as compatible as possible with all wallets, so you don't need to worry about what type of wallet the user has connected. It currently works with Coinbase Smart Wallet, thirdweb in-app wallets, thirdweb smart wallets, and any third party wallets that currently support EIP-5792. In future releases, we'll add fallback support so the wallet reverts to traditional transactions if it doesn't support EIP-5792. Until then, we recommend wrapping sendCalls
in a try
block to catch any errors from unsupported wallets.
Sponsoring transactions
Sponsor your user's transactions with a single line by adding the thirdweb paymaster URL:
You'll be able to configure which domains use this paymaster URL in your thirdweb dashboard. For more complicated paymaster logic, you can setup a paymaster proxy based on ERC-7677.
Get the status of a call bundle
Once you send a set of calls to your wallet, fetch their status using getCallsStatus
. This will return a status
field of either "CONFIRMED" or "PENDING" and a receipts
array containing the completed transaction receipts.
Get a wallet's smart account capabilities
Knowing what UX a wallet can provide helps you surface the right features to your app's users. getCapabilities
returns an object showing the capabilities for different chains the wallet has access to. For thirdweb in-app wallets, this will be the currently connected chain.
Over the coming weeks we'll release additional improvements / support for EIP-5792 and other standards for making dApp development easier than ever.