AI
Response Handling
- Streaming Responses: This method streams data in real-time, providing immediate feedback as the response is generated. Set the
stream
parameter totrue
in your request, the API delivers responses via Server-Sent Events (SSE). - Non-Streaming Responses: When the
stream
parameter is set tofalse
, the API returns the complete response in a single JSON payload after processing is complete.
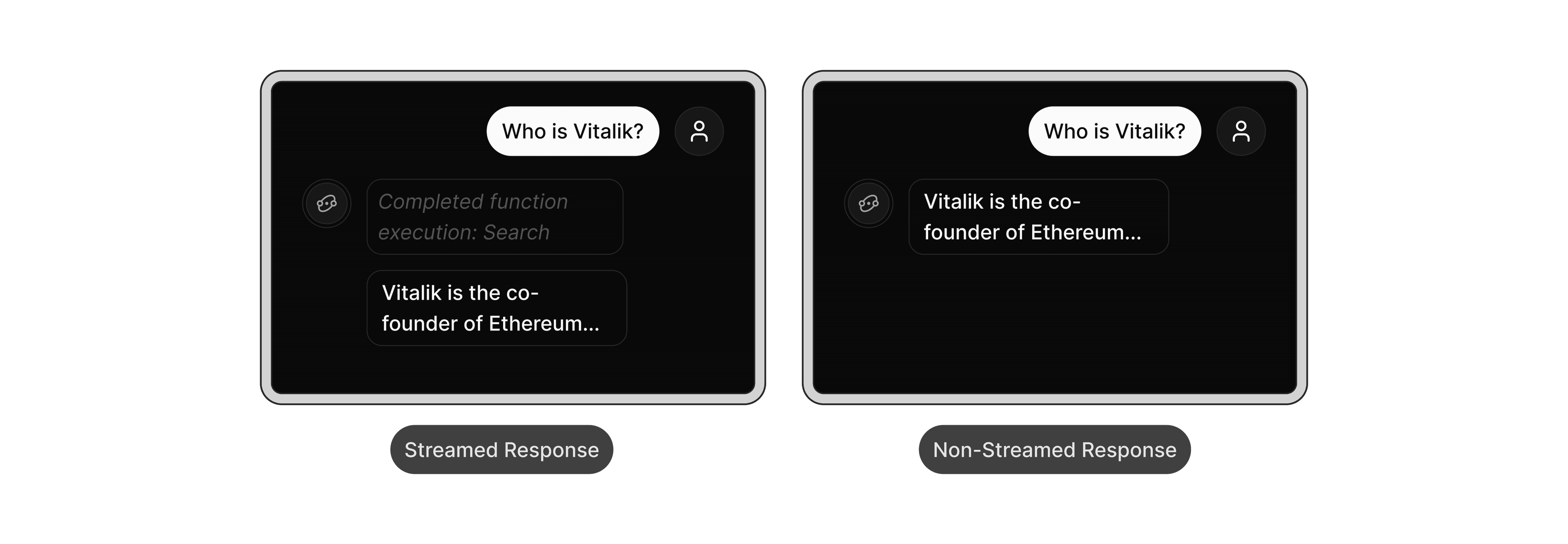
For stream:true
, you'll need to handle the following event types:
init
: Initializes the stream and provides session informationpresence
: Provides backend status updates about worker processingaction
: Contains blockchain transaction or action datadelta
: Contains chunks of the response message texterror
: Contains error information if something goes wrong
Example SSE Stream:
JavaScript Example for handling streams:
By default, responses are returned as JSON Objects. You may optionally specify the desired response format using the response_format
parameter.
- JSON Object: To receive the response as a JSON object, set
response_format
to{ "type": "json_object" }
. - JSON Schema: For responses adhering to a specific JSON schema, set
response_format
to{ "type": "json_schema", "json_schema": { ... } }
, where you define the desired schema structure.
Example with json_schema
format,