Connect Users to your Ecosystem
Ecosystem wallets inherit all the functionality of in-app wallets, but instead of being scoped to a single app, they can be used across multiple applications.
Head over to the thirdweb dashboard to create an ecosystem and obtain your ecosystem id.
Email
Phone
Passkey
Guest
Wallet
Google
Apple
Facebook
X
Discord
Telegram
Twitch
Farcaster
Github
Line
Coinbase
Steam
Backend
Try out ecosystem wallets for yourself in the in-app wallet live playground
The only difference with in-app wallets is how you create an ecosystem wallet using the ecosystemWallet()
function and passing your ecosystem id.
You can configure the allowed auth strategies on your dashboard.
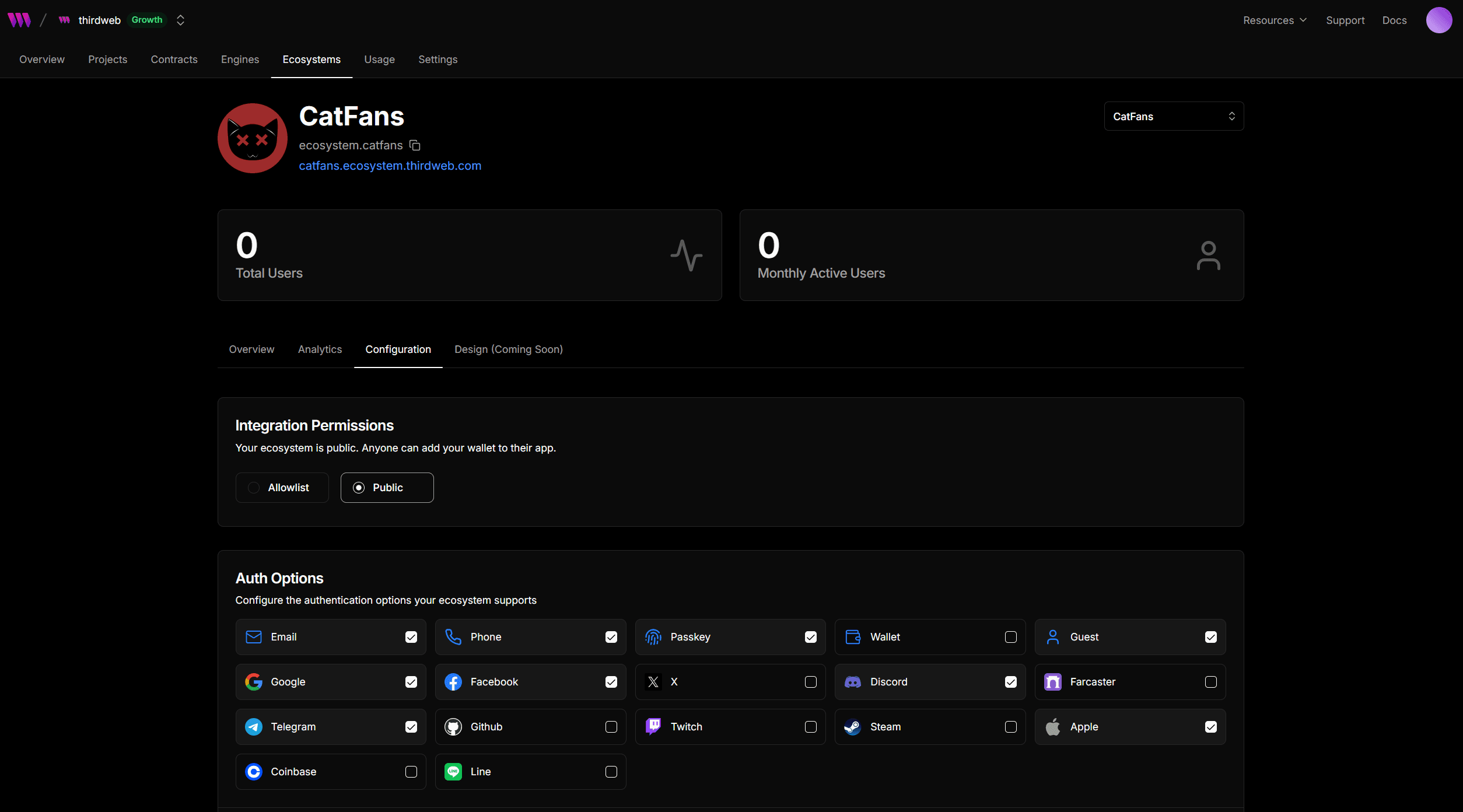
For prebuilt UIs, the enabled auth strategies are automatically read from your dashbaord configurations.
For custom UIs, you can configure the auth options when connecting the wallet. See using your own UI for an example.
Using disabled auth strategies
Note that while you're allowed to pass any auth strategy today, we recommend only passing the strategies that are enabled on your dashboard. We might enforce this in the future.
For closed ecosystems, you can invite partners to your ecosystem. Partners will have to pass a valid partnerId
to the ecosystemWallet()
function in order to connect to your ecosystem.
For more information, refer to the ecosystemWallet API reference.
Refer to the in-app wallets documentation to add your ecosystem wallet to the connect UI components or build your own UI.