ConnectButton
ConnectButton component renders a <button>
which when clicked opens a modal and allows users to connect to various wallets. It is extremely customizable and very easy to use.
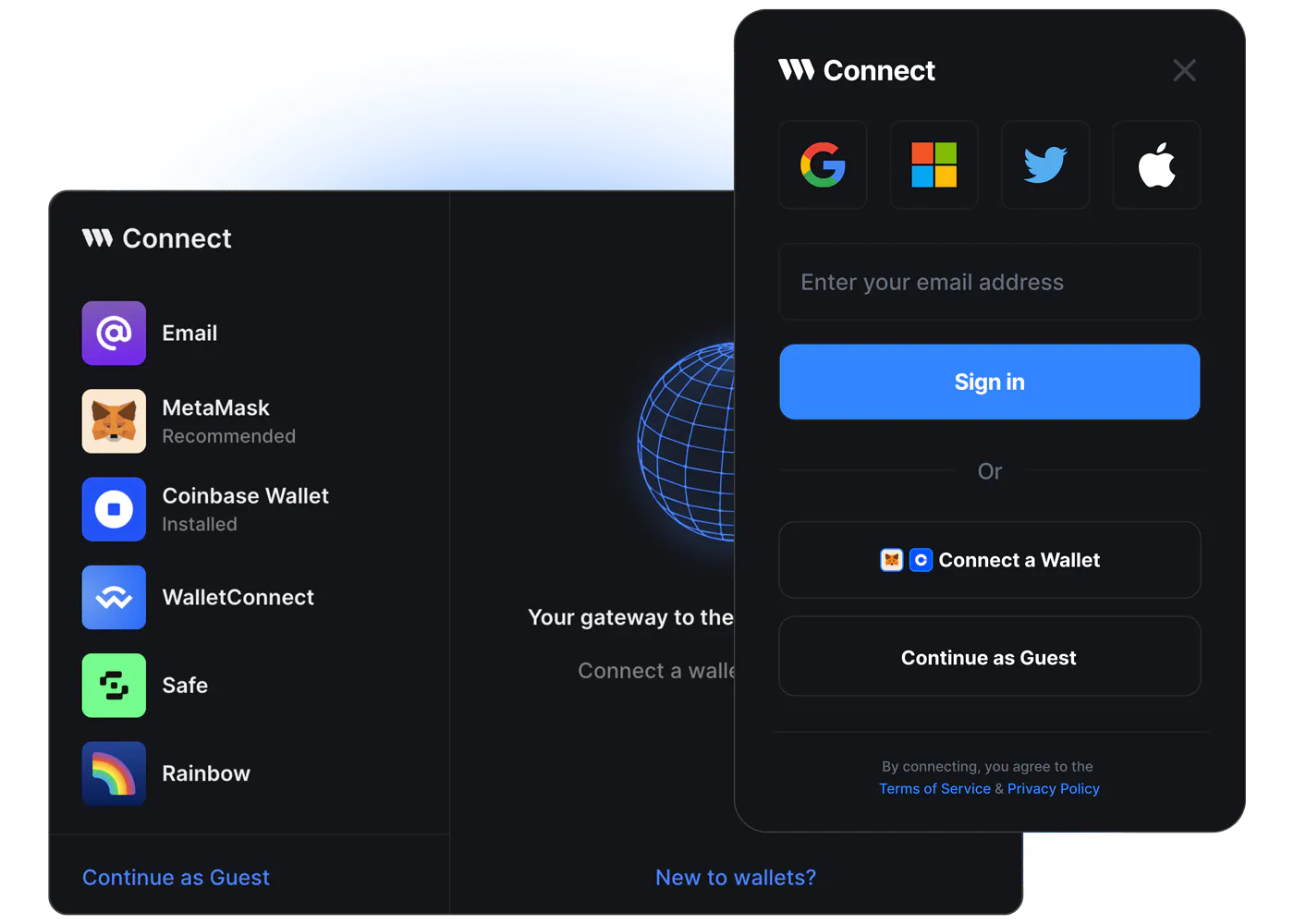
Usage
To Configure the wallets to show in the ConnectButton Modal, configure the wallets
prop
To mark a wallet as Recommended
, pass in the recommended: true
property in the wallet config function.
Refer to Props to see all the available configuration options
Auth
The ConnectButton
can optionally include Auth to manage user authentication for you. When the user connects their wallet, the ConnectButton
will immediately prompt them to sign a message to login. That signature can then be verified on your server to issue the user a JWT authenticating their session.
Each of these functions correspond to a server API endpoint or server-side function. For their implementations, see the TypeScript Auth docs or check out our examples.